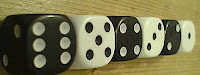
I was thinking about the
PP_NARG macro this morning. It occurred to me that you could use it to create a macro for a range of case labels in a switch statement!
Instead of writing this:
void eg(int n)
{
switch(n)
{
case 1:
case 2:
case 3:
case 4:
case 5:
printf("1..5\n");
break;
}
}
You could write this:
void eg(int n)
{
switch(n)
{
CASES(1,2,3,4,5):
printf("1..5\n");
break;
}
}
and the C99 magic macro is:
#define PP_NARG(...) \
PP_NARG_(__VA_ARGS__, PP_RSEQ_N())
#define PP_NARG_(...) \
PP_ARG_N(__VA_ARGS__)
#define PP_ARG_N(_1, _2, _3, _4, _5, _6, N, ...) N
#define PP_RSEQ_N() \
6,5,4,3,2,1,0
#define CASES(...) \
CASES_(PP_NARG(__VA_ARGS__), __VA_ARGS__)
#define CASES_(N,...) \
CASES_N(N,__VA_ARGS__)
#define CASES_N(N,...) \
CASES_ ## N(__VA_ARGS__)
#define CASES_1(a) case a
#define CASES_2(a,...) case a: CASES_1(__VA_ARGS__)
#define CASES_3(a,...) case a: CASES_2(__VA_ARGS__)
#define CASES_4(a,...) case a: CASES_3(__VA_ARGS__)
#define CASES_5(a,...) case a: CASES_4(__VA_ARGS__)