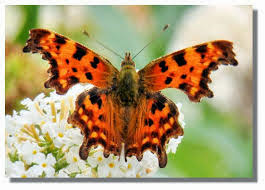
During several cyber-dojos it became clear to me that many of the programmers (despite having programmed in C for several years), did not understand that in C, not all commas are the same. I've created a small piece of C code to try help C programmers understand the humble comma...
Have a look at the following 5 lines of code. Do you know what each line does?
int x = (1,2,3); int x = 1,2,3; x = 1,2,3; x = (1,2,3); x = f(1,2,3);.
.
.
.
.
.
Scroll down for my answers once you've decided...
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
.
int x = (1,2,3);
This declares an
int
called x
and initializes it to the result of the expression
(1,2,3)
. The commas inside this expression are operators.
1 is evaluated and its value (1) discarded,
then a comma provides a sequence point, then 2 is evaluated and its value (2) discarded,
then a comma provides a sequence point, then 3 is evaluted and its value is the value of the
expression (1,2,3)
. So x
is initialized to 3.
You'll probably get warnings saying there are no side-effects in the expressions 1 and 2.
int x = 1,2,3;
This is different. If this compiled it would declare an
int
called x
and initialize it to 1 and then declare two more int
s called 2
and 3
. It has the same structure as int x = 1,y,z;
which declares three
int
s called x
, y
, and z
.
The commas are not operators, they are punctuators/separators.
You can't declare variables called 2
or 3
. It does not compile.
x = 1,2,3;
In this fragment
x
is assumed to have already been declared. It is not a declaration. The
commas are operators again.
Assignment has higher precedence than the comma operator so this binds as (x = 1),2,3;
.
So 1 is assigned to x, and the result of this assignment expression (1) is discarded,
then there is a sequence point, then 2 is evaluated and its value (2) is discarded,
then there is a sequence point, then 3 is evaluated and its value (3) is discarded.
You'll probaby get warnings saying there are no side-effects in the expressions 2 and 3.
x = (1,2,3);
Again,
x
is assumed to have already been declared. It is not a declaration.
The commas are operators again. This is the same as the first fragment except it is not
a declaration. x
is assigned the value of the expression (1,2,3)
which is 3. Again you'll probably get warnings saying there are no side-effects in the expressions 1 and 2.
x = f(1,2,3);
Again,
x
is assumed to have already been declared. As has a function called
f
which accepts three int
arguments. These commas are
not operators. They are punctuators/separators. They separate the three expressions
forming the three arguments to f
. But they do not introduce any sequence points.
How did you do?
No comments:
Post a Comment